In this article, I will discuss the Learning Rust for Solana Development. The Solana blockchain uses Rust as the primary language for smart contracts because of its performance and security features.
For dependable and sturdy decentralized applications, understanding the functionalities of Rust is crucial. In this article, I will explain the initial steps and materials needed to start learning.
What is Rust?
Rust is a contemporary programming language centered on systems development, created with a focus on performance, safety, and simultaneous execution of tasks.
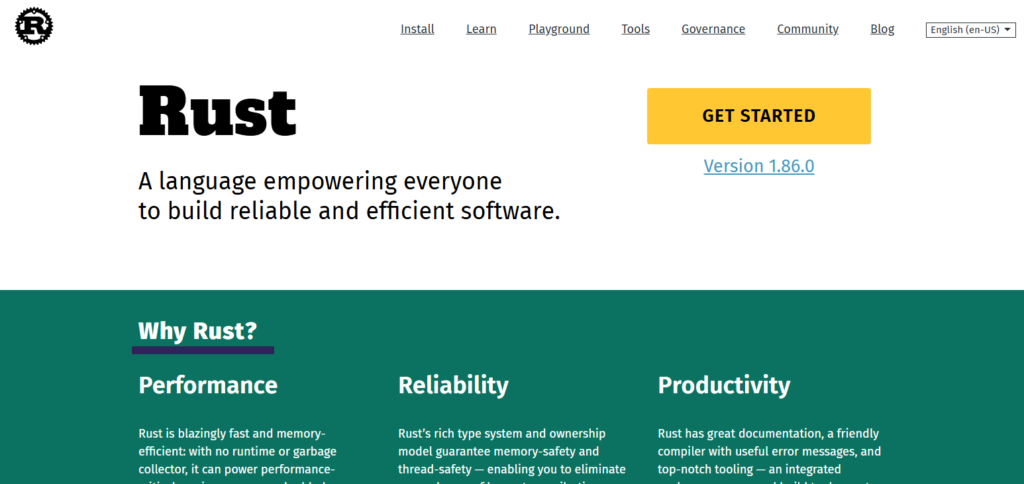
It ensures the safety of memory in building software systems without the aid of garbage collection, making it perfect for constructing dependable and efficient programs. Its use in game engines, operating systems, and web assembly testify to its versatility.
Its ownership structure in Rust allows the prevention of null pointer references or data races, ensures reliability, and checks many forms of software bugs.
Learning Rust For Solana Development
Grasp Rust Fundamentals
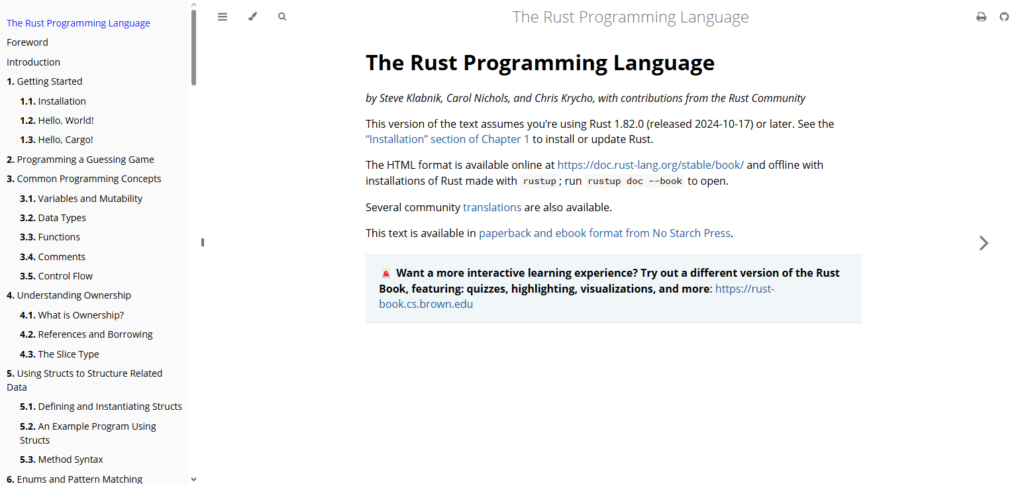
The official Rust book (https://doc.rust-lang.org/book/) should be your starting point as it covers variables, ownership, borrowing, lifetimes, enums and structs, pattern matching, and error handling.
Practice Rust:
Use Exercism or LeetCode to work on problems using Rust to reinforce your understanding of its syntax and logic.
Learn About Cargo and Crates
Make sure to learn about how to use Cargo for building, testing, and managing dependencies, along with exploring useful libraries on Rust’s crate repository, crates.io.
Study Solana Concepts
Familiarize yourself with fundamental concepts of Solana like accounts, programs (smart contracts), instruction sets, transactions, and runtime. The Solana Docs will be your primary source of information.
Set Up The Solana Development Environment
Install Rust using rustup.
Install Solana CLI (sh -c “$(curl -sSfL https://release.solana.com/stable/install)”).
Install anchor (Solana’s Rust-based framework) using cargo install –git https://github.com/coral-xyz/anchor anchor-cli –locked.
Learn Anchor Framework
Check out the documentation regarding Anchor because it adds macros and a structure that simplifies the writing of Solana programs in Rust.
Build a simple program
Create a basic counter or to-do app using Anchor and learn how to define accounts, instructions, and manage state changes.
Deploy and Test on Devnet:
Deploy the program using the Solana CLI, then interact with the Devnet using TypeScript or the CLI.
Explore Advanced Concepts Learn about Cross Program Invocation (CPI), Program Derived Addresses (PDAs), and security best practices.
Contribute or Build Projects
Take part in Solana hackathons, or contribute to open-source Solana projects to acquire hands-on experience.
Core Rust Concepts for Solana Development
Ownership And Borrowing
Rust’s Ownership: enables guarantee on memory access. In Solana programs, ownership denotes what accounts or data structures your program is able to change and read.
Use borrowing (&
) for references to read data without changing it.
Error Handling
Solana programs impose strict control on errors. Manage errors using the Result
type. Return ProgramError
for error codes of specified types.
Memory Management
There is no garbage collection in Rust and instead relies on stack allocation. This manages account data and program state with respect to Solana’s constraints over efficiency.
Modules
Each Solana program should be organized using modules (mod
) to maintain sanity in the code. Group related functions and structures.
Traits
Traits are used to implement shared functional behavior across types. These are used in Solana for standard processes like serialization and deserialization (borsh
or serde
).
Macros
Macros help simplify repeating processes in Solana Programs. This can be defining the entrypoint!
or packing/unpacking pointers in/out of structures.
Data Structures
Typically structured data types are used in solana programs to specify account outline. Use #[derive(BorshSerialize, BorshDeserialize)]
on a struct to enable dealing with on chain accounts.
Lifetimes
Lifetimes guarantee references are valid within a certain range. It is sensitive to context when one works with borrowed data from account arrays.
Concurrency
Solana programs are monotreaded, but inRust testing environments, its concurrency constructs such as “Mutex” or “RwLock” are quite useful.
Custom Types
Create new enums and and structs that will capture the program’s instructions and states. This enables constructing the logic of the program in a clearer way and more rigorously with types.
Why Solana Uses Rust
The usage of Rust programming language can be of great importance for Solana, a blockchain platform, as its development requires careful attention to aspects of speed and safety.
With Rust, smart contracts are more secure because of its memory safety feature which helps eliminate bugs and vulnerabilities.
Furthermore, as stated before, there are no abstractions and high efficiency that allow smooth functioning of Solana’s on-chain programs.
Additionally, robust types in Rust allow easy construction of complex and dependable code which is very helpful regarding Solana.
Best Resources to Learn Rust for Solana
Common Challenges
Understanding Ownership & Borrowing: Rust’s memory model can be pretty complicated for novices. Beginners often misuse references and end up with a myriad of compilation errors.
Account Size Errors: Smaller space
than needed for an account will cause the transaction to fail.
Neglecting the mut
keyword: The absence of mut
on some accounts or variables that need mutation is a common error.
Incorrect PDA Derivation: PDAs need to be exactly the same on the client and program side; otherwise, mismatch signature error will arise.
Serialization Issues: Missing or wrongly defined structs as well as #[account]
alongside #[derive(AnchorSerialize, AnchorDeserialize)]
not defined can prevent deserialization.
Helpful Tips
Use Anchor Framework: Low-level details are abstracted by Anchor and even includes useful macros for account management.
Read Compiler Errors Carefully: Detailed Rust errors enforce inevitability to check compiler errors. Take your time and read them in entirety.
Solana Logs: Use this command to check program logs and debug transactions.
Anchor IDL: Autogenerated IDL can be used for effortless construction of client apps, especially on typescript.
Test Locally: Run anchor test
to conduct local testing before deploying to devnet or mainnet.
Conclusion
Learning Rust for Solana development is a valuable journey that combines the power of a secure, high-performance language with the scalability of one of the fastest blockchain networks.
By mastering Rust’s fundamentals and diving into Solana’s architecture using tools like Anchor, you can build efficient, secure smart contracts. With consistent practice and the right resources, you’ll be well-equipped to develop on Solana confidently.